Planet Python
Last update: June 27, 2025 01:43 AM UTC
June 25, 2025
TestDriven.io
Building a Multi-tenant App with Django
This tutorial looks at how to implement multi-tenancy in Django.
Peter Bengtsson
Native connection pooling in Django 5 with PostgreSQL
Enabling native connection pooling in Django 5 gives me a 5.4x speedup.
Real Python
Your Guide to the Python print() Function
If you’re like most Python users, then you probably started your Python journey by learning about print()
. It helped you write your very own “Hello, World!” one-liner and brought your code to life on the screen. Beyond that, you can use it to format messages and even find some bugs. But if you think that’s all there is to know about Python’s print()
function, then you’re missing out on a lot!
Keep reading to take full advantage of this seemingly boring and unappreciated little function. This tutorial will get you up to speed with using Python print()
effectively. However, be prepared for a deep dive as you go through the sections. You may be surprised by how much print()
has to offer!
By the end of this tutorial, you’ll understand that:
- The
print()
function can handle multiple arguments and custom separators to format output effectively. - You can redirect
print()
output to files or memory buffers using thefile
argument, enhancing flexibility. - Mocking
print()
in unit tests helps verify code behavior without altering the original function. - Using the
flush
argument ensures immediate output, overcoming buffering delays in certain environments. - Thread-safe printing is achievable by implementing locks to prevent output interleaving.
If you’re just getting started with Python, then you’ll benefit most from reading the first part of this tutorial, which illustrates the essentials of printing in Python. Otherwise, feel free to skip ahead and explore the sections that interest you the most.
Get Your Code: Click here to download the free sample code that shows you how to use the print() function in Python.
Take the Quiz: Test your knowledge with our interactive “The Python print() Function” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
The Python print() FunctionIn this quiz, you'll test your understanding of Python's built-in print() function, covering how to format output, specify custom separators, and more.
Printing in a Nutshell
It’s time to jump in by looking at a few real-life examples of printing in Python. By the end of this section, you’ll know every possible way of calling print()
.
Producing Blank Lines
The simplest example of using Python print()
requires just a few keystrokes:
print()
This produces an invisible newline character, which in turn causes a blank line to appear on your screen. To add vertical space, you can call print()
multiple times in a row like this:
print()
print()
print()
It’s just as if you were hitting Enter on your keyboard in a word processor program or a text editor.
While you don’t pass any arguments to print()
, you still need to put empty parentheses at the end of the line to tell Python to actually execute that function rather than just refer to it by name. Without parentheses, you’d obtain a reference to the underlying function object:
>>> print()
>>> print
<built-in function print>
The code snippet above runs within an interactive Python REPL, as indicated by the prompt (>>>
). Because the REPL executes each line of Python code immediately, you see a blank line right after calling print()
. On the other hand, when you skip the trailing parentheses, you get to see a string representation of the print()
function itself.
As you just saw, calling print()
without arguments results in a blank line, which is a line comprised solely of the newline character. Don’t confuse this with an empty string, which doesn’t contain any characters at all, not even the newline!
You can use Python’s string literals to visualize these two:
- Blank Line:
"\n"
- Empty String:
""
The first string literal is exactly one character long, whereas the second one has no content—it’s empty.
Note: To remove the newline character from a string in Python, use its .rstrip()
method, like this:
>>> "A line of text.\n".rstrip()
'A line of text.'
This strips any trailing whitespace from the right edge of the string of characters. To learn more about .rstrip()
, check out the How to Strip Characters From a Python String tutorial.
Even though Python usually takes care of the newline character for you, it helps to understand how to deal with it yourself.
Dealing With Newlines
Read the full article at https://realpython.com/python-print/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
Mike Driscoll
An Intro to ty – The Extremely Fast Python type checker
Ty is a brand new, extremely fast Python type checker written in Rust from the fine folks at Astral, the makers of Ruff. Ty is in preview and is not ready for production use, but you can still try it out on your code base to see how it compares to Mypy or other popular Python type checkers.
Getting Started with ty
You can try out ty using the online playground, or run ty with uvx to get started quickly:
uvx ty
If you prefer to install ty, you can use pip:
python -m pip install ty
Astral provides other installation methods as well.
Using the ty Type Checker
Want to give ty a try? You can run it in much the same way as you would Ruff. Open up your terminal and navigate to your project’s top-level directory. Then run the following command:
ty check
If ty finds anything, you will quickly see the output in your terminal.
Astral has also provided a way to exclude files from type checking. By default, ty ignores files listed in an .ignore
or .gitignore
file.
Adding ty to Your IDE
The Astral team maintains an official VS Code extension for ty. You can get it from the VS Code Marketplace. Their documentation states that other IDEs can also use ty if they support the language server protocol.
Wrapping Up
Ruff is a great tool and has been adopted by many teams since its release. Ty will likely follow a similar trajectory if it as fast and useful as Ruff has been. Only time will tell. However, these new developments in Python tooling are exciting and will be fun to try. If you have used ty, feel free to jump into the comments and let me know what you think.
The post An Intro to ty – The Extremely Fast Python type checker appeared first on Mouse Vs Python.
Real Python
Quiz: The Python print() Function
In this quiz, you’ll test your understanding of Your Guide to the Python print() Function.
The print()
function outputs objects to the console or a specified file-like stream. You’ll practice:
- Printing multiple values with custom separators
- Changing the end-of-line character
- Redirecting output using the
file
parameter - Forcing immediate output with the
flush
parameter
Work through these questions to reinforce your knowledge of print()
’s parameters and best practices for clear, formatted I/O.
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
PyPodcats
Trailer: Episode 9 With Tamara Atanasoska
A preview of our chat with Tamara Atanasoska. Watch the full episode on June 27, 2025A preview of our chat with Tamara Atanasoska. Watch the full episode on June 27, 2025
Sneak Peek of our chat with Tamara Atanasoska, hosted by Georgi Ker and Mariatta Wijaya.
Tamara has been contributing to open source projects since 2012. She participated in Google Summer of Code to contribute to projects like Gnome and e-cidadania.
She is now a maintainer of Fairlearn, an open-source, community-driven project to help data scientists improve fairness of AI systems.
Hear how Django helps her feel empowered, and how the PyLadies Berlin community has helped her feel welcomed as a new immigrant in Germany.
In this episode, Tamara shares perspective about open source contributions, maintain, mentorship, and her experience in running beginner-friendly sprints.
Full episode is coming on June 27, 2025! Subscribe to our podcast now!
Talk Python to Me
#511: From Notebooks to Production Data Science Systems
If you're doing data science and have mostly spent your time doing exploratory or just local development, this could be the episode for you. We are joined by Catherine Nelson to discuss techniques and tools to move your data science game from local notebooks to full-on production workflows.<br/> <br/> <strong>Episode sponsors</strong><br/> <br/> <a href='https://talkpython.fm/agntcy'>Agntcy</a><br> <a href='https://talkpython.fm/sentry'>Sentry Error Monitoring, Code TALKPYTHON</a><br> <a href='https://talkpython.fm/training'>Talk Python Courses</a><br/> <br/> <h2 class="links-heading">Links from the show</h2> <div><strong>New Course: LLM Building Blocks for Python</strong>: <a href="https://training.talkpython.fm/courses/llm-building-blocks-for-python" target="_blank" >training.talkpython.fm</a><br/> <br/> <strong>Catherine Nelson LinkedIn Profile</strong>: <a href="https://www.linkedin.com/in/catherinenelson1/?featured_on=talkpython" target="_blank" >linkedin.com</a><br/> <strong>Catherine Nelson Bluesky Profile</strong>: <a href="https://bsky.app/profile/catnelson.bsky.social?featured_on=talkpython" target="_blank" >bsky.app</a><br/> <strong>Enter to win the book</strong>: <a href="https://forms.gle/1okKtSdSNTtAd4SRA?featured_on=talkpython" target="_blank" >forms.google.com</a><br/> <strong>Going From Notebooks to Scalable Systems - PyCon US 2025</strong>: <a href="https://us.pycon.org/2025/schedule/presentation/51/?featured_on=talkpython" target="_blank" >us.pycon.org</a><br/> <strong>Going From Notebooks to Scalable Systems - Catherine Nelson – YouTube</strong>: <a href="https://www.youtube.com/watch?v=o4hyA4hotxw&ab_channel=PyConUS" target="_blank" >youtube.com</a><br/> <strong>From Notebooks to Scalable Systems Code Repository</strong>: <a href="https://github.com/catherinenelson1/from_notebooks_to_scalable?featured_on=talkpython" target="_blank" >github.com</a><br/> <strong>Building Machine Learning Pipelines Book</strong>: <a href="https://www.oreilly.com/library/view/building-machine-learning/9781492053187/?featured_on=talkpython" target="_blank" >oreilly.com</a><br/> <strong>Software Engineering for Data Scientists Book</strong>: <a href="https://www.oreilly.com/library/view/software-engineering-for/9781098136192/?featured_on=talkpython" target="_blank" >oreilly.com</a><br/> <strong>Jupytext - Jupyter Notebooks as Markdown Documents</strong>: <a href="https://github.com/mwouts/jupytext?featured_on=talkpython" target="_blank" >github.com</a><br/> <strong>Jupyter nbconvert - Notebook Conversion Tool</strong>: <a href="https://github.com/jupyter/nbconvert?featured_on=talkpython" target="_blank" >github.com</a><br/> <strong>Awesome MLOps - Curated List</strong>: <a href="https://github.com/visenger/awesome-mlops?featured_on=talkpython" target="_blank" >github.com</a><br/> <strong>Watch this episode on YouTube</strong>: <a href="https://www.youtube.com/watch?v=n2WFfVIqlDw" target="_blank" >youtube.com</a><br/> <strong>Episode #511 deep-dive</strong>: <a href="https://talkpython.fm/episodes/show/511/from-notebooks-to-production-data-science-systems#takeaways-anchor" target="_blank" >talkpython.fm/511</a><br/> <strong>Episode transcripts</strong>: <a href="https://talkpython.fm/episodes/transcript/511/from-notebooks-to-production-data-science-systems" target="_blank" >talkpython.fm</a><br/> <br/> <strong>--- Stay in touch with us ---</strong><br/> <strong>Subscribe to Talk Python on YouTube</strong>: <a href="https://talkpython.fm/youtube" target="_blank" >youtube.com</a><br/> <strong>Talk Python on Bluesky</strong>: <a href="https://bsky.app/profile/talkpython.fm" target="_blank" >@talkpython.fm at bsky.app</a><br/> <strong>Talk Python on Mastodon</strong>: <a href="https://fosstodon.org/web/@talkpython" target="_blank" ><i class="fa-brands fa-mastodon"></i>talkpython</a><br/> <strong>Michael on Bluesky</strong>: <a href="https://bsky.app/profile/mkennedy.codes?featured_on=talkpython" target="_blank" >@mkennedy.codes at bsky.app</a><br/> <strong>Michael on Mastodon</strong>: <a href="https://fosstodon.org/web/@mkennedy" target="_blank" ><i class="fa-brands fa-mastodon"></i>mkennedy</a><br/></div>
June 24, 2025
PyCoder’s Weekly
Issue #687: Scaling With Kubernetes, Substrings, Big-O, and More (June 24, 2025)
#687 – JUNE 24, 2025
View in Browser »
Scaling Web Applications With Kubernetes and Karpenter
What goes into scaling a Python web application today? What are resources for learning and practicing DevOps skills? This week on the show, Calvin Hendryx-Parker is back to discuss the tools and infrastructure for autoscaling web applications with Kubernetes and Karpenter.
REAL PYTHON podcast
The Fastest Way to Detect a Vowel in a String
If you need to find the vowels in a string there are several different approaches you could take. This article covers 11 different ways and how each performs.
AUSTIN Z. HENLEY
Prevent Postgres Slowdowns on Python Apps with this Check List
Avoid performance regressions in your Python app by staying on top of Postgres maintenance. This monthly check list outlines what to monitor, how to catch slow queries early, and ways to ensure indexes, autovacuum, and I/O are performing as expected →
PGANALYZE sponsor
O(no) You Didn’t
A deep dive into why real-world performance often defies Big-O expectations and why context and profiling matter more than theoretical complexity
MRSHINY608
Discussions
Python Jobs
Sr. Software Developer (Python, Healthcare) (USA)
Articles & Tutorials
The PSF’s 2024 Annual Impact Report Is Here!
The Python Software Foundation releases a report every year on the state of the PSF and our community. This year’s report gives a run down of the successes of the language, key highlights of PyCon US, updates from the developers in residence, and more.
PYTHON SOFTWARE FOUNDATION
Are Python Dictionaries Ordered Data Structures?
Although dictionaries have maintained insertion order since Python 3.6, they aren’t strictly speaking ordered data structures. Read on to find out why and how the edge cases can be important depending on your use case.
STEPHEN GRUPPETTA
10 Polars Tools and Techniques to Level Up Your Data Science
Are you using Polars for your data science work? There are many libraries out there that might help you write less code. Talk Python interviews Christopher Trudeau and they talk about the Polars ecosystem.
KENNEDY & TRUDEAU podcast
Cut Django DB Latency With Native Connection Pooling
“Deploy Django 5.1’s native connection pooling in 10 minutes to cut database latency by 50-70ms, reduce connection overhead by 60-80%, and improve response times by 10-30% with zero external dependencies.”
SAURABH KUMAR
Will AI Replace Junior Developers?
At PyCon US this year, Adarsh chatted with various Python folks about one big question: will AI replace junior developers? He spoke with Guido van Rossum, Anthony Shaw, Simon Willison, and others.
ADARSH DIVAKARAN • Shared by Adarsh Divakaran
PEP 779: Free-Threaded Python (Accepted)
Free-threaded Python has been upgraded from experimental to part of the supported build. This quick quote from a longer discussion covers exactly what that means.
SIMON WILSON
PSF Board Election Schedule
It is time for the Python Software Foundation Board elections. Nominations are due by July 29th. See the article for the full election schedule and deadlines.
PYTHON SOFTWARE FOUNDATION
Exploring Python’s list
Data Type With Examples
In this video course, you’ll dive deep into Python’s lists: how to create them, update their content, populate and grow them - with practical code examples.
REAL PYTHON course
Write Pythonic and Clean Code With namedtuple
Discover how Python’s namedtuple
lets you create simple, readable data structures with named fields you can access using dot notation.
REAL PYTHON
Execute Your Python Scripts With a Shebang
In this video course, you’ll learn when and how to use the shebang line in your Python scripts to execute them from a Unix-like shell.
REAL PYTHON course
All About the TypedDict
Are you fan of type hinting in Python? Learn how to add typing to a dictionary with different types of keys.
MIKE DRISCOLL
Projects & Code
Events
Weekly Real Python Office Hours Q&A (Virtual)
June 25, 2025
REALPYTHON.COM
PyCamp Leipzig 2025
June 28 to June 30, 2025
BARCAMPS.EU
Launching Python Katsina Community
June 28 to June 29, 2025
PYTHONKATSINA.ORG
PyDelhi User Group Meetup
June 28, 2025
MEETUP.COM
Workshop: Creating Python Communities
June 29 to June 30, 2025
PYTHON-GM.ORG
PyCon Colombia 2025
July 4 to July 7, 2025
PYCON.CO
Happy Pythoning!
This was PyCoder’s Weekly Issue #687.
View in Browser »
[ Subscribe to 🐍 PyCoder’s Weekly 💌 – Get the best Python news, articles, and tutorials delivered to your inbox once a week >> Click here to learn more ]
Real Python
Starting With DuckDB and Python
The DuckDB database provides a seamless way to handle large datasets in Python with Online Analytical Processing (OLAP) optimization. You can create databases, verify data imports, and perform efficient data queries using both SQL and DuckDB’s Python API.
By the end of this video course, you’ll understand that:
- You can create a DuckDB database by reading data from files like Parquet, CSV, or JSON and saving it to a table.
- You query a DuckDB database using standard SQL syntax within Python by executing queries through a DuckDB connection object.
- You can also use DuckDB’s Python API, which uses method chaining for an object-oriented approach to database queries.
- Concurrent access in DuckDB allows multiple reads but restricts concurrent writes to ensure data integrity.
- DuckDB integrates with pandas and Polars by converting query results into DataFrames using the
.df()
or.pl()
methods.
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
June 23, 2025
Real Python
Python enumerate(): Simplify Loops That Need Counters
Python’s enumerate()
function helps you with loops that require a counter by adding an index to each item in an iterable. This is particularly useful when you need both the index and value while iterating, such as listing items with their positions. You can also customize the starting index with the start
argument, offering flexibility in various scenarios.
By the end of this tutorial, you’ll understand that:
enumerate()
in Python adds a counter to an iterable and returns it as anenumerate
object, pairing each item with an index.enumerate()
accepts an iterable and an optionalstart
argument to set the initial index value.- The advantage of
enumerate()
is that it provides both index and value pairs without manual counter management. - You can use
zip()
or slicing as alternatives toenumerate()
for iterating multiple sequences or selecting specific elements.
Explore how to implement your own version of enumerate()
and discover alternative methods like zip()
and itertools
for more complex iteration patterns.
Get Your Code: Click here to download the free sample code you’ll use to explore how enumerate() works in Python.
Using Python’s enumerate()
There are situations when you need both the index and the value of items in an iterable. That’s when Python’s enumerate()
function can come in handy for you.
Python’s enumerate()
function adds a counter to an iterable and returns it in the form of an enumerate
object, which can be used directly in loops. For example, when you want to display the winners of a race, you might write:
>>> runners = ["Lenka", "Martina", "Gugu"]
>>> for winner in enumerate(runners):
... print(winner)
...
(0, 'Lenka')
(1, 'Martina')
(2, 'Gugu')
Since enumerate()
is a built-in function, you can use it right away without importing it. When you use enumerate()
in a for
loop, you get pairs of count and value from the iterable.
Note: If you’re coming from languages like C, Java, or JavaScript, you might be tempted to use range(len())
to get both the index and value in a loop. While this works in Python, enumerate()
is considered a more Pythonic and preferred approach.
In the example above, you’re storing the position and the runner’s name in a variable named winner
, which is a tuple
. To display the position and the name right away, you can unpack the tuple
when you define the for
loop:
>>> runners = ["Lenka", "Martina", "Gugu"]
>>> for position, name in enumerate(runners):
... print(position, name)
...
0 Lenka
1 Martina
2 Gugu
Just like the winner
variable earlier, you can name the unpacked loop variables whatever you want. You use position
and name
in this example, but they could just as easily be named i
and value
, or any other valid Python names.
When you use enumerate()
in a for
loop, you typically tell Python to use two variables: one for the count and one for the value itself. You’re able to do this by applying a Python concept called tuple unpacking.
Unpacking is the idea that a tuple—or another type of a Python sequence—can be split into several variables depending on the length of that sequence. For instance, you can unpack a tuple of two elements into two variables:
>>> cart_item = ("Wireless Mouse", 2)
>>> product_name, quantity_ordered = cart_item
>>> product_name
'Wireless Mouse'
>>> quantity_ordered
2
First, you create a tuple with two elements, "Wireless Mouse"
and 2
. Then, you unpack that tuple into product_name
and quantity_ordered
, which are each assigned one of the values from the tuple.
When you call enumerate()
and pass a sequence of values, Python returns an iterator. When you ask the iterator for its next value, it yields a tuple with two elements. The first element of the tuple is the count, and the second element is the value from the sequence that you passed:
>>> values = ["first", "second"]
>>> enumerate_instance = enumerate(values)
>>> print(enumerate_instance)
<enumerate at 0x7fe75d728180>
>>> next(enumerate_instance)
(0, 'first')
>>> next(enumerate_instance)
(1, 'second')
>>> next(enumerate_instance)
Traceback (most recent call last):
File "<python-input-5>", line 1, in <module>
next(enumerate_instance)
~~~~^^^^^^^^^^^^^^^
StopIteration
In this example, you create a Python list called values
with two elements, "first"
and "second"
. Then, you pass values
to enumerate()
and assign the return value to enumerate_instance
. When you print enumerate_instance
, you’ll see that it’s an enumerate
object with a particular memory address.
Then, you use Python’s built-in next()
to get the next value from enumerate_instance
. The first value that enumerate_instance
returns is a tuple with the count 0
and the first element from values
, which is "first"
.
Calling next()
again on enumerate_instance
yields another tuple—this time, with the count 1
and the second element from values
, "second"
. Finally, calling next()
one more time raises a StopIteration
exception since there are no more values to be returned from enumerate_instance
.
When an iterable is used in a for
loop, Python automatically calls next()
at the start of every iteration until StopIteration
is raised. Python assigns the value it retrieves from the iterable to the loop variable.
By default, the count of enumerate()
starts at 0
. This isn’t ideal when you want to display the winners of a race. Luckily, Python’s enumerate()
has one additional argument that you can use to control the starting value of the count.
When you call enumerate()
, you can use the start
argument to change the starting count:
>>> runners = ["Lenka", "Martina", "Gugu"]
>>> for position, name in enumerate(runners, start=1):
... print(position, name)
...
1 Lenka
2 Martina
3 Gugu
In this example, you pass start=1
, which initializes position
with the value 1
on the first loop iteration.
You should use enumerate()
anytime you need to use the count and an item in a loop. Keep in mind that enumerate()
increments the count by one on every iteration. However, this only slightly limits your flexibility. Since the count is a standard Python integer, you can use it in many ways.
Practicing With Python enumerate()
Now that you’ve explored the basics of enumerate()
, it’s time to practice using enumerate()
with some real-world examples.
Read the full article at https://realpython.com/python-enumerate/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
Daniel Roy Greenfeld
TIL: HTML 404 errors for FastAPI
I had a TIL about custom responses in FastAPI but there's enough nuance that it deserves a full blog post. In the meantime, here's a TIL about custom HTTP responses.
from fastapi import FastAPI
from fastapi.responses import HTMLResponse
async def custom_404_exception_handler(request, exc):
return HTMLResponse(
f'<p>404 Not Found at "{request.url.path}"</p>', status_code=404
)
# Add more HTTP exceptions as needed
HTTP_EXCEPTIONS = {404: custom_404_exception_handler}
app = FastAPI(exception_handlers=HTTP_EXCEPTIONS)
@app.get("/")
async def read_root():
return {"Hello": "World"}
Try it out by running the app and going to a non-existent path, like /not-found
. You should see a simple HTML page with a 404 message.
Python Bytes
#437 Python Language Summit 2025 Highlights
<strong>Topics covered in this episode:</strong><br> <ul> <li><em>* <a href="https://pyfound.blogspot.com/2025/06/python-language-summit-2025.html?featured_on=pythonbytes">The Python Language Summit 2025</a></em>*</li> <li><strong><a href="https://willmcgugan.github.io/fixing-python-properties/?featured_on=pythonbytes">Fixing Python Properties</a></strong></li> <li><em>* <a href="https://github.com/rohaquinlop/complexipy?featured_on=pythonbytes">complexipy</a></em>*</li> <li><em>* <a href="https://github.com/OKUA1/juvio?featured_on=pythonbytes">juvio</a></em>*</li> <li><strong>Extras</strong></li> <li><strong>Joke</strong></li> </ul><a href='https://www.youtube.com/watch?v=sOJf3iBM6Tg' style='font-weight: bold;'data-umami-event="Livestream-Past" data-umami-event-episode="437">Watch on YouTube</a><br> <p><strong>About the show</strong></p> <p><strong>Sponsored by Posit:</strong> <a href="https://pythonbytes.fm/connect">pythonbytes.fm/connect</a></p> <p><strong>Connect with the hosts</strong></p> <ul> <li>Michael: <a href="https://fosstodon.org/@mkennedy">@mkennedy@fosstodon.org</a> / <a href="https://bsky.app/profile/mkennedy.codes?featured_on=pythonbytes">@mkennedy.codes</a> (bsky)</li> <li>Brian: <a href="https://fosstodon.org/@brianokken">@brianokken@fosstodon.org</a> / <a href="https://bsky.app/profile/brianokken.bsky.social?featured_on=pythonbytes">@brianokken.bsky.social</a></li> <li>Show: <a href="https://fosstodon.org/@pythonbytes">@pythonbytes@fosstodon.org</a> / <a href="https://bsky.app/profile/pythonbytes.fm">@pythonbytes.fm</a> (bsky)</li> </ul> <p>Join us on YouTube at <a href="https://pythonbytes.fm/stream/live"><strong>pythonbytes.fm/live</strong></a> to be part of the audience. Usually <strong>Monday</strong> at 10am PT. Older video versions available there too.</p> <p>Finally, if you want an artisanal, hand-crafted digest of every week of the show notes in email form? Add your name and email to <a href="https://pythonbytes.fm/friends-of-the-show">our friends of the show list</a>, we'll never share it.</p> <p><strong>Michael #1: <a href="https://pyfound.blogspot.com/2025/06/python-language-summit-2025.html?featured_on=pythonbytes">The Python Language Summit 2025</a></strong></p> <ul> <li>Write up by Seth Michael Larson</li> <li><a href="https://pyfound.blogspot.com/2025/06/python-language-summit-2025-how-can-we-make-breaking-changes-less-painful.html?featured_on=pythonbytes">How can we make breaking changes less painful?</a>: talk by Itamar Oren</li> <li><a href="https://pyfound.blogspot.com/2025/06/python-language-summit-2025-uncontentious-talk-about-contention.html?featured_on=pythonbytes">An Uncontentious Talk about Contention</a>: talk by Mark Shannon</li> <li><a href="https://pyfound.blogspot.com/2025/06/python-language-summit-2025-state-of-free-threaded-python.html?featured_on=pythonbytes">State of Free-Threaded Python</a>: talk by Matt Page</li> <li><a href="https://pyfound.blogspot.com/2025/06/python-language-summit-2025-fearless-concurrency.html?featured_on=pythonbytes">Fearless Concurrency</a>: talk by Matthew Parkinson, Tobias Wrigstad, and Fridtjof Stoldt</li> <li><a href="https://pyfound.blogspot.com/2025/06/python-language-summit-2025-challenges-of-the-steering-council.html?featured_on=pythonbytes">Challenges of the Steering Council</a>: talk by Eric Snow</li> <li><a href="https://pyfound.blogspot.com/2025/06/python-language-summit-2025-docs-editorial-board.html?featured_on=pythonbytes">Updates from the Python Docs Editorial Board</a>: talk by Mariatta</li> <li><a href="https://pyfound.blogspot.com/2025/06/python-language-summit-2025-packaging-governance-process.html?featured_on=pythonbytes">PEP 772 - Packaging Governance Process</a>: talk by Barry Warsaw and Pradyun Gedam</li> <li><a href="https://pyfound.blogspot.com/2025/06/python-language-summit-2025-python-on-mobile.html?featured_on=pythonbytes">Python on Mobile - Next Steps</a>: talk by Russell Keith-Magee</li> <li><a href="https://pyfound.blogspot.com/2025/06/python-language-summit-2025-what-do-core-developers-want-from-rust.html?featured_on=pythonbytes">What do Python core developers want from Rust?</a>: talk by David Hewitt</li> <li><a href="https://pyfound.blogspot.com/2025/06/python-language-summit-upstreaming-the-pyodide-js-ffi.html?featured_on=pythonbytes">Upstreaming the Pyodide JS FFI</a>: talk by Hood Chatham</li> <li><a href="https://pyfound.blogspot.com/2025/06/python-language-summit-2025-lightning-talks.html?featured_on=pythonbytes">Lightning Talks</a>: talks by Martin DeMello, Mark Shannon, Noah Kim, Gregory Smith, Guido van Rossum, Pablo Galindo Salgado, and Lysandros Nikolaou</li> </ul> <p><strong>Brian #2:</strong> <a href="https://willmcgugan.github.io/fixing-python-properties/?featured_on=pythonbytes">Fixing Python Properties</a></p> <ul> <li>Will McGugan</li> <li>“Python properties work well with type checkers such Mypy and friends. … The type of your property is taken from the getter only. Even if your setter accepts different types, the type checker will complain on assignment.”</li> <li>Will describes a way to get around this and make type checkers happy.</li> <li>He replaces <code>@property</code> with a descriptor. It’s a cool technique.</li> <li>I also like the way Will is allowing different ways to use a property such that it’s more convenient for the user. This is a cool deverloper usability trick.</li> </ul> <p><strong>Brian #3: <a href="https://github.com/rohaquinlop/complexipy?featured_on=pythonbytes">complexipy</a></strong></p> <ul> <li>Calculates the cognitive complexity of Python files, written in Rust.</li> <li>Based on the cognitive complexity measurement described in a white paper by Sonar</li> <li>Cognitive complexity builds on the idea of cyclomatic complexity.</li> <li>Cyclomatic complexity was intended to measure the “testability and maintainability” of the control flow of a module. Sonar argues that it’s fine for testability, but doesn’t do well with measuring the “maintainability” part. So they came up with a new measure.</li> <li>Cognitive complexity is intended to reflects the relative difficulty of understanding, and therefore of maintaining methods, classes, and applications.</li> <li>complexipy essentially does that, but also has a really nice color output.</li> <li>Note: at the very least, you should be using “cyclomatic complexity” <ul> <li>try with <code>ruff check --select C901</code></li> </ul></li> <li>But also try complexipy.</li> <li>Great for understanding which functions might be ripe for refactoring, adding more documentation, surrounding with more tests, etc.</li> </ul> <p><strong>Michael #4: <a href="https://github.com/OKUA1/juvio?featured_on=pythonbytes">juvio</a></strong></p> <ul> <li>uv kernel for Jupyter</li> <li>⚙️ <strong>Automatic Environment Setup:</strong> When the notebook is opened, Juvio installs the dependencies automatically in an ephemeral virtual environment (using <code>uv</code>), ensuring that the notebook runs with the correct versions of the packages and Python</li> <li>📁 <strong>Git-Friendly Format:</strong> Notebooks are converted on the fly to a script-style format using <code># %%</code> markers, making diffs and version control painless</li> <li>Why Use Juvio? <ul> <li>No additional lock or requirements files are needed</li> <li>Guaranteed reproducibility</li> <li>Cleaner Git diffs</li> </ul></li> <li>Powered By <ul> <li><code>uv</code> – ultra-fast Python package management</li> <li><code>PEP 723</code> – Python inline dependency standards</li> </ul></li> </ul> <p><strong>Extras</strong></p> <p>Brian:</p> <ul> <li><a href="https://testandcode.com?featured_on=pythonbytes">Test & Code</a> in slow mode currently. But will be back with some awesome interviews.</li> </ul> <p><strong>Joke: <a href="https://www.youtube.com/watch?v=hwG89HH0VcM">The 0.1x Engineer</a></strong></p> <ul> <li>via Balázs</li> <li>Also <ul> <li><a href="https://www.youtube.com/watch?v=NY2nnsAplSo&ab_channel=StormtrooperVlogs">StormTrooper vlog</a></li> <li><a href="https://www.youtube.com/watch?v=oHYaOdIyuQA">BIGFOOT VLOG - ATTACKED BY WENDIGO!</a></li> </ul></li> </ul>
June 21, 2025
Will McGugan
Seeking sponsorship for Rich and Textual
After a somewhat stressful three years running a startup, I am takings a year's sabbatical. I'm going to use this time to focus on my health‐something I have neglected. As good as it feels not to work full-time, I still plan on maintaining Rich, Textual and other Open Source projects, in addition to offering free tech support.
Since I don't have any income at the moment, I want to start looking for sponsorship again. I'm hoping it will rise to a level where it will cover living costs for the year, but I'll be happy if it pays for coffee.
If you have benefited from my work in the past, consider sponsoring me. Whatever you feel comfortable with.
If your organization has extracted any value from my work, then consider picking one of the higher tiers to return the favor. I may be able to assist you with your project, wether it is terminal related or not. There is a lot of knowledge in my fleshy mammalian brain. Even in the age of AI, it could still benefit you.
Armin Ronacher
My First Open Source AI Generated Library
I'm currently evaluating how different models perform when generating XML versus JSON. Not entirely unexpectedly, XML is doing quite well — except for one issue: the models frequently return invalid XML. That made it difficult to properly assess the quality of the content itself, independent of how well the models serialize data. So I needed a sloppy XML parser.
After a few failed attempts of getting Claude to just fix up my XML parsing in different ways (it tried html5lib, the html lxml parser etc.) which all resulted in various kinds of amusing failures, I asked Claude to ultrathink and write me a proper XML library from scratch. I gave it some basic instructions of what this should look like and it one-shotted something. Afterwards I prompted it ~20 more times to do various smaller fixes as a response to me reviewing it (briefly) and using it and to create an extensive test suite.
While that was taking place I had 4o create a logo. After that I quickly converted it into an SVG with Illustrator and had Claude make it theme-aware for dark and light modes, which it did perfectly.
On top of that, Claude fully set up CI and even remotely controlled my browser to configure the trusted PyPI publisher for the package for me.
In summary, here is what AI did:
- It wrote ~1100 lines of code for the parser
- It wrote ~1000 lines of tests
- It configured the entire Python package, CI, PyPI publishing
- Generated a README, drafted a changelog, designed a logo, made it theme-aware
- Did multiple refactorings to make me happier
The initial prompt that started it all (including typos):
I want you to implement a single-file library here that parses XML sloppily. It should implement two functions:
- stream_parse which yields a stream of events (use named tuples) for the XML stream
- tree_parse which takes the output of stream_parse and assembles an element tree. It should default to xml.etree.ElementTree and optoinally allow you to provide lxml too (or anything else)
It should be fast but use pre-compiled regular expressions to parse the stream. The idea here is that the output comes from systems that just pretend to speak XML but are not sufficiently protected against bad outoput (eg: LLMs)
So for instance & should turn into & but if &x is used (which is invalid) it should just retain as &x in the output. Additionally if something is an invalid CDATA section we just gracefully ignore it. If tags are incorrectly closed within a larger tag we recover the structure. For instance <foo><p>a<p>b</foo> will just close the internal structures when </foo> comes around.
Use ultrathink. Break down the implementation into
- planning
- api stubs
- implementation
Use sub tasks and sub agents to conserve context
Now if you look at that library, you might not find it amazingly beautiful. It probably is a bit messy and might have a handful of bugs I haven't found yet. It however works well enough for me right now for what I'm doing and it definitely unblocked me. In total it worked for about 30-45 minutes to write the initial implementation while I was doing something else. I kept prompting it for a little while to make some progress as I ran into issues using it.
If you want to look at what it looks like:
To be clear: this isn't an endorsement of using models for serious Open Source libraries. This was an experiment to see how far I could get with minimal manual effort, and to unstick myself from an annoying blocker. The result is good enough for my immediate use case and I also felt good enough to publish it to PyPI in case someone else has the same problem.
Treat it as a curious side project which says more about what's possible today than what's necessarily advisable.
Postscriptum: Yes, I did slap an Apache 2 license on it. Is that even valid when there's barely a human in the loop? A fascinating question but not one I'm not eager to figure out myself. It is however certainly something we'll all have to confront sooner or later.
June 20, 2025
The Python Coding Stack
I Want to Remove Duplicates from a Python List • How Do I Do It?
Another short article today to figure out ways to remove duplicate values from a list. The ideal solution depends on what you really need.
Let's explore.
Start With a List
Well, we need a list first–ideally, one with duplicate values. So, let's assume we have an online queue (line). But some people put their name in the queue more than once:

Note how James and Kate were eager to ensure they were in the queue, so they put their name down twice.
Removing Duplicates: The Ugly Way
I was initially tempted not to include this section, but I changed my mind, as you can see. You can come up with several algorithms to perform this task "manually". It's only a few lines of code. Here's one option:
You have a queue_unique
empty list ready to collect unique names. Next, you iterate using enumerate()
and add names to queue_unique
if they don't appear in the rest of the original list. Note that I'm using slicing in the if
statement to slice the list from index + 1
to the end of the list.
Let me show you another option. I'll discuss the outputs from these two manual versions later in this article:
This time, you reverse the list so you can loop through the names in reverse order. The queue_unique
doesn't start as an empty list this time but as a copy of the original reversed list.
In the loop, you remove names from queue_unique
if the name appears later in the reversed list. A reminder that the .remove()
list method only removes the first occurrence of an item. It doesn't remove all of them.
Both algorithms remove duplicates. Great. But compare the output from the two versions. The difference between these output lists gives a clue to what's coming next.
But I won't dwell on these versions any longer.
and PS: there are better versions of manual algorithms for this, but that's not the point of this first section, so let's move on!
Removing Duplicates: The Set Way
When you learn about data structures, you learn about the various characteristics they have. Then, you start comparing data structures based on these characteristics. For example, lists, dictionaries, tuples, and strings are all iterable. But lists and dictionaries are mutable, whereas tuples and strings are immutable. And lists, tuples, and strings are all sequences, but dictionaries are not–they're mappings. You can read more about some of these categories here: The Python Data Structure Categories Series
And some data structures enforce uniqueness while others don't. Lists, as you've seen above, can have several equal items–in the example above, you have several strings that are equal to each other.
However, sets are a Python data structure that can only have unique values:
So, the easiest way to remove duplicates from a list is to cast it into a set:
Or, if you prefer the output to still be a list, and perhaps you also want to overwrite the original variable name, then you can write the following:
Now, that was easy! Much better than the several lines of code in the previous section.
However, there's an issue. If this is a queue of customers, then the order in which they joined the queue is somewhat important, I would say!
Note how the new queue
list, the one without duplicates, no longer maintains the original order of the people within it. James was the first to join the queue, but Andy appears to have moved to the front when you removed duplicates.
Note that this also happened with the first of the manual algorithms in the previous section.
Sometimes, you don't care about the order of the elements in a list. If that's the case, you can cast the list into a set and then back into a list to remove duplicates.
But sometimes, the order matters. It certainly matters when dealing with a queue of customers. Let's look at another option.
Removing Duplicates: The Dictionary Way
A quick question before I carry on. Did you read the latest article I published just before this one? Here's the link: Are Python Dictionaries Ordered Data Structures?
If you haven't, now is a good time to read it. Like this one, it's a short article, so it won't take you too long.
You're back. Great.
So, you now know that since Python 3.7, there's a guarantee that the order of insertion of items in a dictionary is maintained. And dictionary keys must also be unique–you cannot have the same key appear twice in a dictionary.
Therefore, if you could create a dictionary from the elements in the list queue
, you would remove duplicates but also maintain the order. And there's a dictionary class method for that:
You create a dictionary from the list queue
. The items in the list become keys, and each key has a default value of None
. You can customise this default value, but you don't need to in this case, as you'll see in the next paragraph.
Great, you removed duplicates while maintaining order since dictionaries maintain order. The dictionary is created by iterating through the list, which explains why this version maintains the order of the items. But you don't want a dictionary, and you don't care about the values within it. So, you can cast this dictionary back into a list. You only keep the keys when you cast a dictionary into a list:
You've now removed duplicates from the list and maintained the original order by converting the list into a dictionary and then back into a list.
Simple–once you know this idiom.
Do you want to join a forum to discuss Python further with other Pythonistas? Upgrade to a paid subscription here on The Python Coding Stack to get exclusive access to The Python Coding Place's members' forum. More Python. More discussions. More fun.
And you'll also be supporting this publication. I put plenty of time and effort into crafting each article. Your support will help me keep this content coming regularly and, importantly, will help keep it free for everyone.
A Limitation
Both the set and dictionary routes have an important limitation. Items in a set must be hashable objects. And keys in a dictionary must also be hashable. Therefore, you can't use these techniques if you have a list that includes non-hashable objects, such as a list that contains other lists.
You can read more about hashability and hashable objects in this post: Where's William? How Quickly Can You Find Him? • What's a Python Hashable Object?
Final Words
You may need to remove duplicates from a list in Python.
Don't write your own algorithm. Life's too short for that.
If you don't care about the order of the items in the list, cast the list into a set and then back into a list: list(set(queue))
If you do care about the order, create a dictionary from the list using dict.fromkeys()
and then cast it back into a list: list(dict.fromkeys(queue))
.
And the set and dictionary routes to removing duplicates are also more efficient than the manual ones shown above. So, it’s a win-win.
That's it.
Photo by Lisett Kruusimäe: https://www.pexels.com/photo/flowers-in-line-on-white-background-9510861/
Code in this article uses Python 3.13
The code images used in this article are created using Snappify. [Affiliate link]
You can also support this publication by making a one-off contribution of any amount you wish.
For more Python resources, you can also visit Real Python—you may even stumble on one of my own articles or courses there!
Also, are you interested in technical writing? You’d like to make your own writing more narrative, more engaging, more memorable? Have a look at Breaking the Rules.
And you can find out more about me at stephengruppetta.com
Further reading related to this article’s topic:
Appendix: Code Blocks
Code Block #1
queue = ["James", "Kate", "Andy", "James", "Isabelle", "Kate"]
Code Block #2
queue_unique = []
for index, name in enumerate(queue):
if name not in queue[index + 1:]:
queue_unique.append(name)
queue_unique
# ['Andy', 'James', 'Isabelle', 'Kate']
Code Block #3
queue = ['James', 'Kate', 'Andy', 'James', 'Isabelle', 'Kate']
queue.reverse()
queue
# ['Kate', 'Isabelle', 'James', 'Andy', 'Kate', 'James']
queue_unique = queue.copy()
for index, name in enumerate(queue):
if name in queue[index + 1:]:
queue_unique.remove(name)
queue_unique.reverse()
queue_unique
# ['James', 'Kate', 'Andy', 'Isabelle']
Code Block #4
set([1, 2, 3, 4, 3, 2, 1])
# {1, 2, 3, 4}
Code Block #5
queue = ["James", "Kate", "Andy", "James", "Isabelle", "Kate"]
set(queue)
# {'Andy', 'James', 'Kate', 'Isabelle'}
Code Block #6
queue = list(set(queue))
queue
# ['Andy', 'James', 'Kate', 'Isabelle']
Code Block #7
queue = ["James", "Kate", "Andy", "James", "Isabelle", "Kate"]
dict.fromkeys(queue)
# {'James': None, 'Kate': None, 'Andy': None, 'Isabelle': None}
Code Block #8
queue = list(dict.fromkeys(queue))
queue
# ['James', 'Kate', 'Andy', 'Isabelle']
For more Python resources, you can also visit Real Python—you may even stumble on one of my own articles or courses there!
Also, are you interested in technical writing? You’d like to make your own writing more narrative, more engaging, more memorable? Have a look at Breaking the Rules.
And you can find out more about me at stephengruppetta.com
Ruslan Spivak
Book Notes: Full Frontal Calculus by Seth Braver — Chapter 1 Review
“Where there is life, there is change; where there is change, there is calculus.” — Seth Braver
I recently went back to studying math to rebuild my foundations for AI and machine learning. I didn’t expect to enjoy a calculus book this much. Shocking, I know. But that’s exactly what happened with Full Frontal Calculus.
Can calculus feel intuitive? Even fun? From the first few pages? For me, the answer was yes. (Okay, from page 8 to be exact.)
Why This Book Clicked for Me
As part of my self-study, I’m reviewing select chapters from the books I work through. This post covers Chapter 1 of Full Frontal Calculus by Seth Braver.
Before I stumbled on Full Frontal Calculus, I tried a few limit-based calculus books and textbooks, but none of them spoke to me. Luckily, there’s no shortage of calculus material these days, so it’s easy to shop around and try different sources.
Braver’s book grabbed me right away. The early focus on infinitesimals, the tight writing, and the emphasis on intuition won me over. I even caught myself smiling more than once. Rare for a math book.
Chapter 1 Highlights
Chapter 1 starts with infinitesimals: “an infinitely small number, smaller than any positive real number, yet greater than zero.” One early example shows how a circle, imagined as a polygon with infinitely many infinitesimal sides, leads to the familiar area formula πr². If your geometry or trig is rusty, don’t worry - it still makes sense. Braver then uses the same idea to show how curves appear straight on a small enough (infinitesimal) scale, which is the heart of differential calculus.
Things really clicked for me in the section titled A Gift From Leibniz: d-Notation. Braver’s explanation of dy/dx shows how it captures infinitesimal change in a way that just makes sense. It helped me understand why derivatives represent slopes and rates in a way I could explain to a 10-year-old. Working through the derivative of x² from first principles was also deeply satisfying.
Practically speaking, Chapter 1 covers:
- what infinitesimals are
- how they help us define rates of change
- the geometric meaning of derivatives
- the elegant dy/dx notation from Leibniz
- why we ignore higher-order infinitesimals like (dx)² or du * dv
- and a first-principles derivation of the derivative of x²
The chapter ends with two powerful tools: the power rule and linearity properties. These let you compute derivatives of polynomials using just basic mental math.
The writing is sharp and often funny, in a math kind of way. There’s even a cameo by the Sumerian beer goddess Ninkasi, who helps explain rate of change and derivatives using a vat of beer. It sounds quirky, but it works.
The book’s style, clarity, and focus on intuition made me want to keep going. That’s not something I’ve felt with many math books.
Final Thoughts and Tips
If you’re following along or just curious about studying calculus again, I recommend giving Chapter 1 a shot. It’s not always light reading, and the exercises are essential, but it might click for you like it did for me. Chapter 1 is available for free on the author’s site, so you can explore it before deciding whether to dive in.
If you do decide to dive into the book, here are a few tips to get the most out of it:
- If you’re rusty on pre-calculus (I was), make sure you’ve got slope, rate of change, the point-slope formula, and the slope-intercept form down cold before the Rates of Change section on page 10. For that, Seth Braver’s other book Precalculus Made Difficult has excellent material on those topics. You can probably get through it in a day.
- Read slowly, with a pen or pencil in hand. Write in the margins (get a paperback copy). It might feel painfully slow at times (pun intended), but it’s a recipe for deeper understanding.
- The book includes answers to many exercises and is great for self-study. But the solutions are compact, so I recommend using Grok or ChatGPT to expand on them and deepen your understanding.
- Once you’ve finished the chapter and exercises, check out the author’s YouTube videos that go along with the book. They’re criminally underrated and oddly hard to find. You might enjoy them as much as I do.
- For topics that are hard to retain, try spaced repetition with active recall. Anki works great for that, or use whatever tool you prefer.
Chapter 1 sealed the deal. This is the calculus book I’m sticking with. Looking forward to seeing how Braver develops the ideas from here.
More to come. Stay tuned.
Originally published in my newsletter Beyond Basics. If you’d like to get future posts like this by email, you can subscribe here.
P.S. I’m not affiliated with the author. I just really enjoy the book and wanted to share it.
Real Python
The Real Python Podcast – Episode #254: Scaling Python Web Applications With Kubernetes and Karpenter
What goes into scaling a web application today? What are resources for learning and practicing DevOps skills? This week on the show, Calvin Hendryx-Parker is back to discuss the tools and infrastructure for autoscaling web applications with Kubernetes and Karpenter.
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
June 19, 2025
EuroPython
June Newsletter: Last Chance for Tickets!
Hello, Pythonistas! 🐍
We added a lot of new subscribers since the last newsletter – if this is your first newsletter – Welcome! 🎉
TL;DR:
- Some of the tickets are sold out already 🎉
- We have a Python documentary premiere at EuroPython
- Memorial session for Michael Foord
- New sprints venue, and a completely new Social Event on an island in the heart of Prague this year!
- Community Organisers & PyLadies Events
- Speaker guidelines and an update on the Speaker Mentorship Programme
- Snacks exchange
- And a surprise at the end of the newsletter below
⏰ Tickets
We’re excited to share that tutorial and combined tickets are now sold out!
Conference tickets are still available – but don’t wait too long. Late Bird pricing kicks in on June 27, and prices will go up! If you can’t attend in person please check our Remote tickets – those are already available in the tickets store.
More details at https://europython.eu/tickets
Platinum, Gold and Silver Sponsorship packages are now fully booked. If you’re interested in sponsoring, please contact us at sponsoring@europython.eu. We’d love to explore options with you! We’ve also added a new startup tier – contact us for more details 🙂
🎥 Documentary on the History of Python
The filmmakers from Cult Repo, formerly known as Honeypot, are working on a documentary about the history of Python and its vibrant community. It features over 20 core developers and takes us on a journey from the first days of Python to the latest developments.
At EuroPython, we’re excited to share a special preview of the film, followed by a Q&A with Brett Cannon, Paul Everitt, and Armin Ronacher.
🖤 Memorial session for Michael Foord
As part of EuroPython, we will be holding a memorial session to commemorate Michael Foord.
Michael Foord (1974-2025) was a central figure in the Python community. He was an original thinker whose warmth, passion, and unfiltered humor touched the lives of many. A core Python developer and the creator of the influential unittest.mock module, he played a pivotal role in shaping testing practices and helped establish the Language Summit at PyCon. More than a brilliant engineer, Michael was a beloved mentor and friend, known for his elaborate vaping setup, colorful attire, and heartfelt conversations. His passing earlier this year left a profound void in the community, but his legacy lives on through his contributions to Python, his generous spirit, and the countless moments of camaraderie he inspired.
Friends of Michael are invited to attend this session and share their memories. We will provide more details about it closer to the event.
© All rights reserved by Nicholas Tollervey | © All rights reserved by Kushal Das |
❣️Beginners’ Day
On Saturday 19th July, we’ll be hosting a Beginners’ Day to help introduce people to Python programming and its applications. Beginners’ Day will feature three tracks running in parallel; The Unconference, Django Girls, and Humble Data. The events are designed to welcome newcomers to the Python ecosystem, including a series of talks and panels by junior developers and two workshops designed to introduce complete beginners to web development and data science.
We are running the following three tracks:
- The Unconference, a series of panels and discussions designed to help people just getting into tech to start or grow their career
- Django Girls, a hands-on workshop teaching the basics of web development
- Humble Data, a hands-on workshop teaching the basics of data science
Beginners’ Day is open to everyone, and you don’t need a EuroPython ticket to attend (although note that some tracks will cost €5 to attend otherwise). From students to those exploring a career change, we warmly invite anyone curious about starting their programming journey. Expect a friendly, fun, and supportive environment that will leave you feeling more confident and inspired to continue learning.
Please see this page for more details and to apply. Places are limited and will be given on a first come, first serve basis.
👩💻 Contribute, collaborate, code: sprints weekend
Join us for EuroPython&aposs traditional Sprint Weekend on Saturday and Sunday (19–20 July) following the main conference. The conference team provides space, lunch, and coffee—you bring the projects, energy, and ideas. Whether you’re a seasoned maintainer or trying your first contribution, sprints are informal hackathons to collaborate on open‑source, share knowledge, and solve problems together.
More info: europython.eu/sprints
🏖️ Pack your instruments, sportswear, or board games
We’ll host a laid‑back social evening on Thursday, 17 July at 19:30 CEST on Střelecký Island—right in the heart of Prague. Expect riverside seating, live music and jam sessions (feel free to bring an instrument), plus board games and plenty of relaxation spots. There&aposs also a mix of outdoor sports (volleyball, croquet, pétanque) and light snacks and drinks for a summery, informal vibe.
A limited number of social-event tickets will be available separately—keep an eye out so you don’t miss out.
More info: europython.eu/social-event
👥 Community Organisers & PyLadies Events
The Python community is an essential part of the language, and for many people, it’s the reason they stick around and keep meetups, conferences, forums, and so much more running to help others.
We have several activities focused on communities across Europe and around the world, as well as initiatives centered around Python itself.
https://europython.eu/community-activities/
We’re excited to announce a range of events for underrepresented groups in computing this year! 🎉 Whether you’re new to PyLadies or a long-time supporter, we warmly welcome you to join us and be part of our supportive community.
These events are open only to those who have a conference ticket, giving our participants an opportunity to connect, share, and grow together.
https://europython.eu/pyladies/
🍬 Snacks exchange
Have you ever wondered what people snack on in Spain? Or wanted to try chocolates from Australia? Then participate in the EuroPython snack exchange!
Simply bring snacks typical of your home country, country of residence, or just a country you think has really delicious food with you to EuroPython. At the conference you’ll be able to swap what you brought with other participants in the exchange. Don’t miss your chance to discover your new favourite snack, and share in the fun with our attendees from across Europe and the globe!
🎤 Speaker guidelines
We’ve uploaded a number of suggestions to help you to prepare your session. The guidelines include information about:
- The audio and technical equipment in each session room
- The capacity of each room
- The time available for each session
- How to share your session slides with attendees on Pretalx
- How to test your equipment on the day and access the Speaker Ready Room
- How to make effective, accessible presentations
- Specific things needed to prepare for tutorial and poster sessions.
👉 Check them out here: https://ep2025.europython.eu/guidelines/
🎤 Speaker Mentorship Programme
First Time Speakers’ Workshop
We had such a fun, interactive session—thank you to everyone who showed up. A huge thank you to Cristián Maureira-Fredes from the Programme team for walking us through the details of giving a talk at EuroPython. We also loved hearing from Iryna Kondrashchenko, who shared how much last year’s Speaker Mentorship Programme helped her speaking journey.
A huge shoutout to our inspiring panel—Abigail Mesrenyame Dogbe, Laís Carvalho, and Rodrigo Girão Serrão. Thank you for sharing your personal experiences as speakers, answering the questions, and offering honest and encouraging advice.
🎥 Missed it? You can watch the recording here: https://youtu.be/a2ZajKY6bm0
❓What does Guido van Rossum like about EuroPython?
And what about speakers, core developers, and other community members? Find out by following us on YouTube and social media! We&aposre sharing short clips where community members talk about what they’re most excited for at the next EuroPython.
- TikTok: https://www.tiktok.com/@europython
- YouTube: https://www.youtube.com/@EuroPythonConference
- Instagram: https://www.instagram.com/europython/
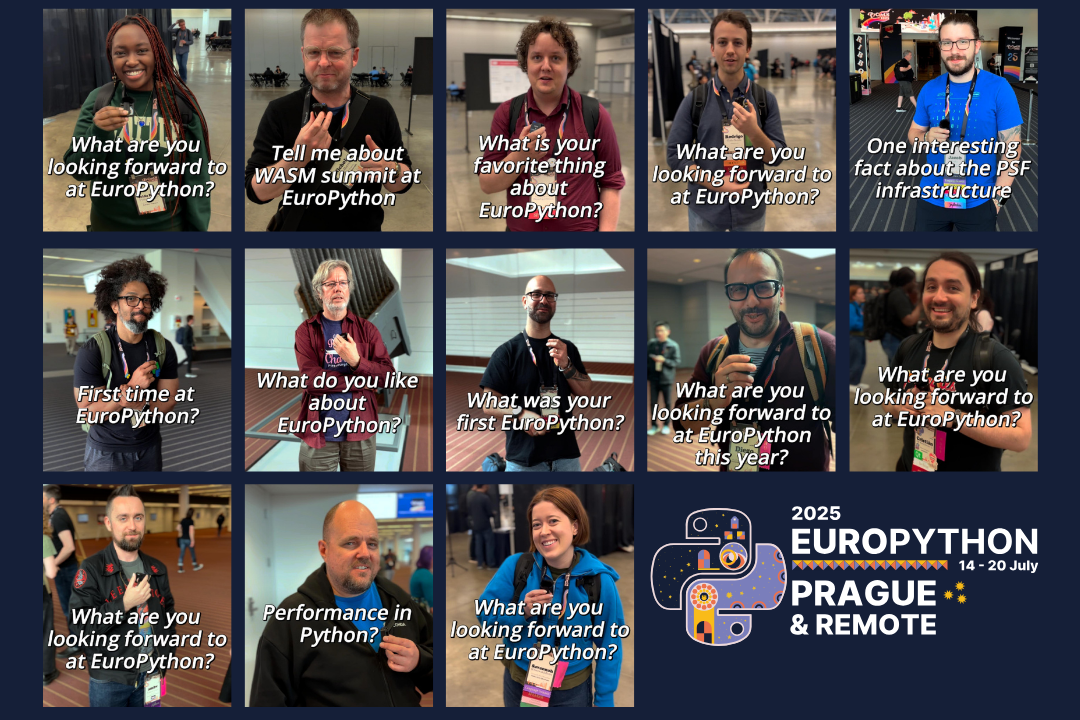
💰Sponsors Highlight
We would like to thank our sponsors for supporting the conference. Their generous contributions help us keep the event more accessible and ticket prices lower. Sponsors play a vital role in making this community gathering possible.
Special thanks go our platinum sponsors:
💞 Upcoming Events in the Python Community
- EuroPython, Prague, 14-20 July https://ep2025.europython.eu
- EuroSciPy, Kraków, 18-22 August https://euroscipy.org/2025/
- PyCon Poland, Gliwice, 28-31 August https://pl.pycon.org/2025/en/
- PyCon Greece 2025, Athens, Greece, 29-30 August https://2025.pycon.gr/en/
- PyCamp CZ 25 beta, Třeštice, 12-14 September https://pycamp.cz/
- Pycon UK, Manchester, 19-22 September https://2025.pyconuk.org/
- PyCon Estonia, Tallinn, 2-3 October https://pycon.ee/
- PyCon Spain, Seville, 17-19 October https://2025.es.pycon.org/
- PyCon Finland, Jyväskylä, 17 October https://2025.ploneconf.org/pyconfi-2025
- PyCon Sweden, Stockholm, 30-31 October https://pycon.se/
- PyCon France, Lyon, 30 October-2 November https://www.pycon.fr/2025/en/index.html
👋 See You All Next Month
Enjoyed this update? Help us spread the word! Like, share, and subscribe — and don’t forget to tell your friends about us.
Someone shared this with you? Join the list at blog.europython.eu to get these directly every month.
Think others in your Python circle would be interested? Forward the email and share it with them. 🙂
Stay connected with us on social media:
- LinkedIn: https://www.linkedin.com/company/europython/
- X: https://x.com/europython
- TikTok: https://www.tiktok.com/@europython
- Mastodon: https://fosstodon.org/@europython
- BlueSky: https://bsky.app/profile/europython.eu
- YouTube: https://www.youtube.com/@EuroPythonConference
- Instagram: https://www.instagram.com/europython/
PyCharm
Training Your ML Models With Cadence
In the rapidly evolving domains of machine learning (ML) and artificial intelligence (AI), the tools and technologies used by developers can significantly influence the speed, efficiency, and effectiveness of their projects. Recognizing this, we introduced Cadence in PyCharm 2025.1, a plugin that merges the ease of local development with advanced cloud computing capabilities.
Why Cadence?
Cadence makes it possible to run your code on powerful cloud hardware directly from PyCharm. This integration alleviates the typical complexities and extensive setup usually associated with cloud computing. Whether you’re a solo developer experimenting with new models or part of a larger team pushing the boundaries of ML applications, Cadence ensures that your transition to powerful cloud resources is seamless and straightforward.
Serverless computing on demand
Reduce overhead with Cadence’s serverless computing options, allowing you to access and manage GPUs with transparent and predictable per-second billing. This removes the need for significant upfront investments in hardware, making advanced computing power accessible at any scale.
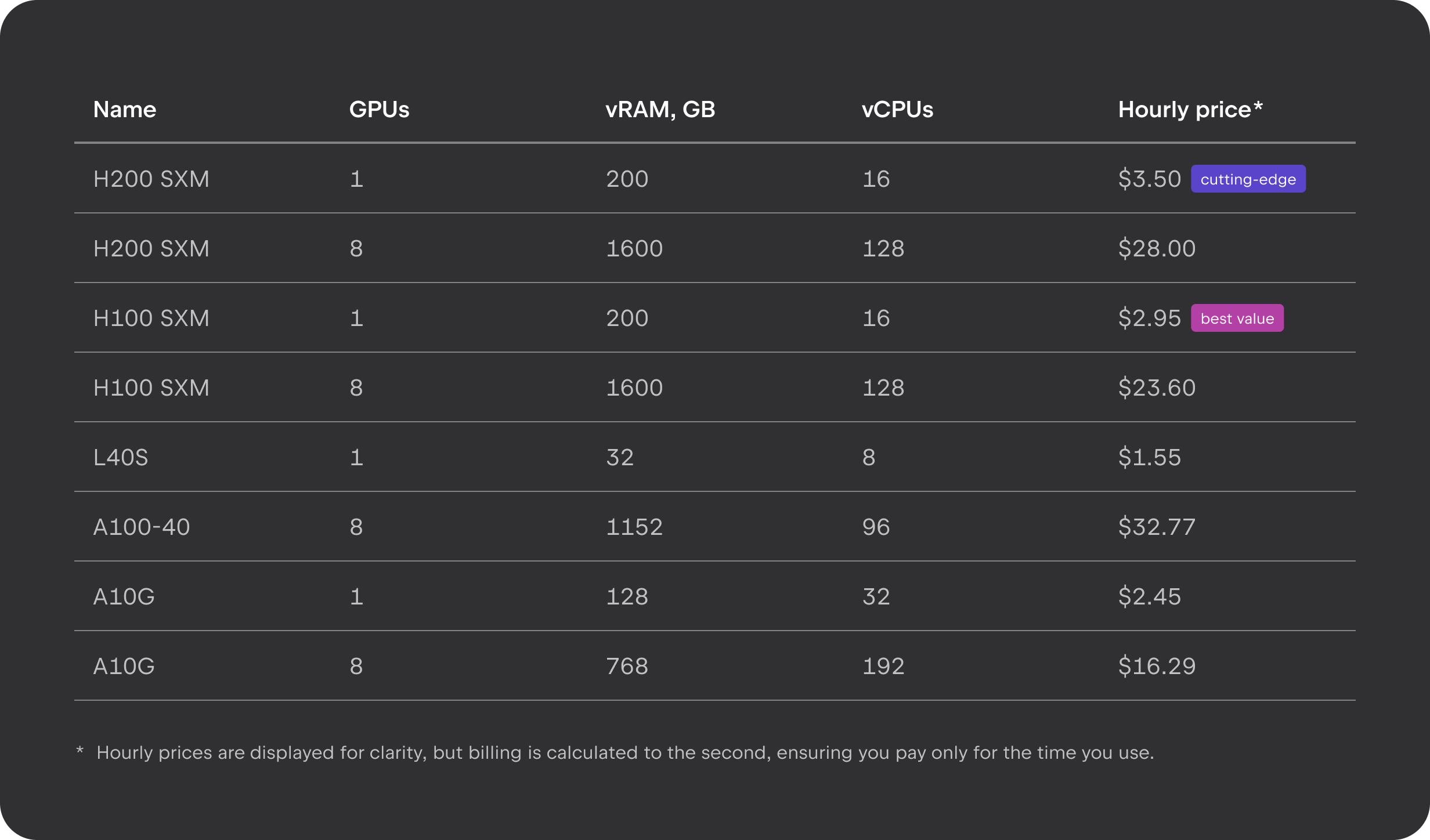
Run your code as is
With Cadence, your existing PyCharm projects require no modifications to fit into the cloud environment. Upload and execute your code as usual; Cadence handles all of the adjustments on the back end, ensuring your cloud session feels like an extension of your local setup.
Tailored for PyCharm users
Debug and deploy using the PyCharm interface you’re familiar with. Set breakpoints, monitor outputs, and interact with your remote environment with no additional learning curve.
Data management simplified
Say goodbye to manual data transfers. Cadence automatically synchronizes your projects’ data to the cloud, allowing you to download the results of each experiment directly in the IDE.
Reliable experimentation
Review, refine, and rerun your past experiments. Cadence provides consistent replication of results, facilitating continuous improvements.
Optimized resource allocation
Choose from a wide array of cloud settings, including configurations like 8xA100 and 8xH100, to scale your resources according to project demands. Schedule as many tasks as you need simultaneously, and Cadence will automatically check for available hosts in different regions and zones.
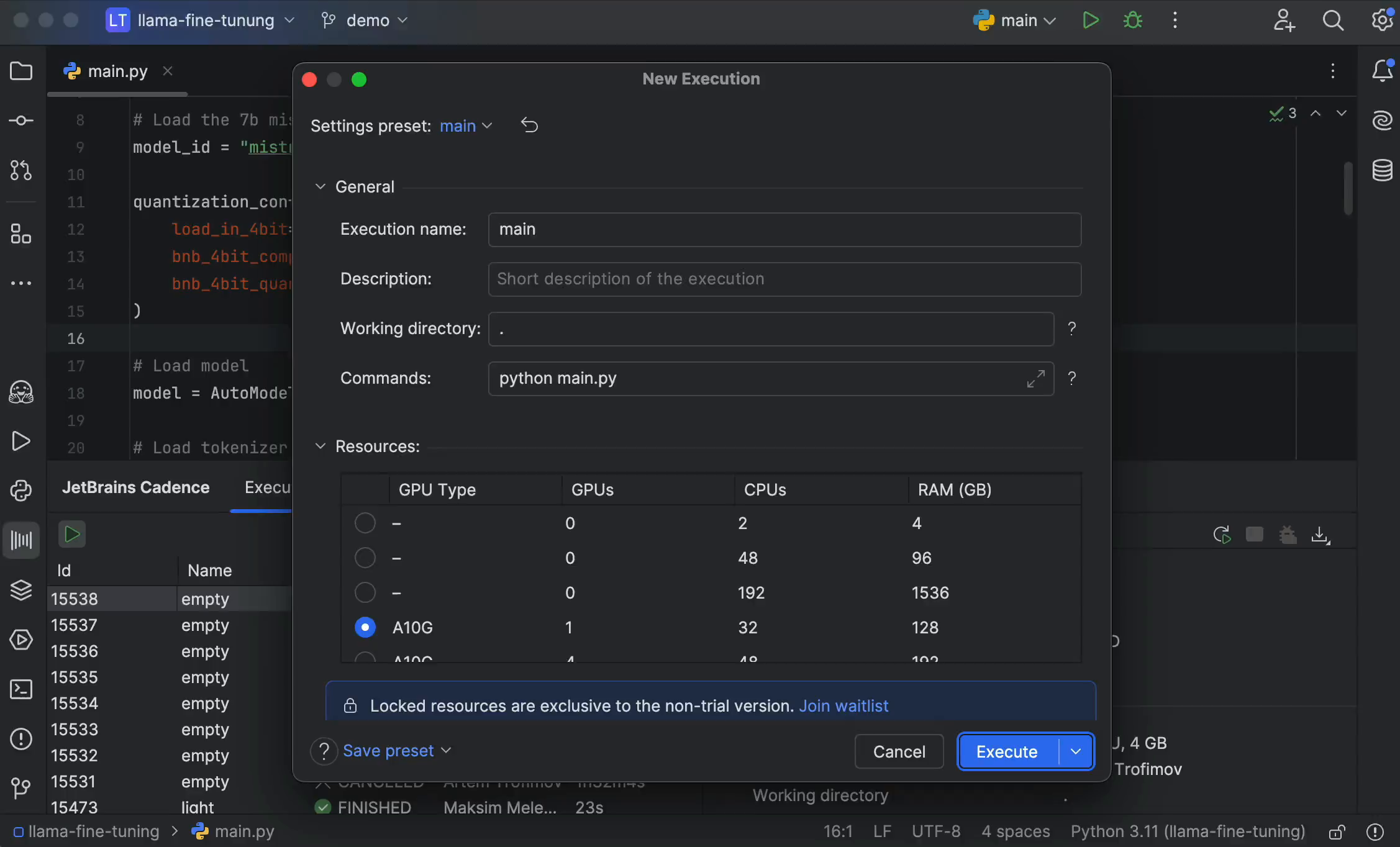
Ready for teams
Adopting Cadence isn’t just about improving individual productivity; it’s about enhancing team dynamics and output. Share setup configurations, results, and insights effortlessly within your team.
Getting started with Cadence
You can try Cadence for free with a USD 30 welcome credit by installing the plugin from JetBrains Marketplace or by enabling it directly in PyCharm via Settings | Plugins | Marketplace.
To see how easy it is to start training your ML models in PyCharm, check out this tutorial video.
June 18, 2025
Talk Python Blog
New Theme Song: Served In A Flask
Those of you who were early listeners of Talk Python To Me might remember the amazing theme song we launched with: Developers, Developers, Developers by Smixx. Thanks to Smixx for letting us use his music for our intros.
Over the years, people have asked “What happened to the rap song”? I took it down for a couple of reasons not worth digging into but have definitely missed the fun and irreverant intro to the show.
Real Python
Python Project: Build a Word Count Command-Line App
The word count command (wc
) is a classic utility that you might use to determine the number of lines, words, and bytes in files or standard input. It’s a staple tool for anyone working with text files on Unix-like systems. But have you ever wondered how such a tool is designed and implemented?
In this practice exercise, you’ll dive into the inner workings of the Unix wc
command by building its simplified version from scratch using Python. Not only will this coding challenge solidify your understanding of file handling and text processing, but it’ll also give you a taste of how to structure command-line utilities in Python.
By the end of this challenge, you’ll have a functional version of the wc
command that can faithfully reproduce the outputs you’re accustomed to seeing in a Unix terminal. However, it won’t be an exact replica of the wc
command, as you’ll omit or adapt some features for simplicity.
In this coding challenge, you’ll:
- Read content from files and standard input (stdin)
- Count the number of lines, words, and bytes in the input
- Differentiate between bytes and characters to handle Unicode
- Implement command-line arguments to specify what counts to display
While working on this challenge, you’ll gain hands-on experience with several modules from Python’s standard library, such as pathlib
for manipulating the file system and argparse
for parsing command-line arguments. Familiarity with basic Python programming and file handling will be beneficial, but detailed instructions and helpful tips will guide you through each step of the process.
The challenge is broken down into a number of tasks, each accompanied by clear instructions and illustrative examples. You’ll receive automated feedback on your solutions when you follow along using the accompanying materials. If you run into any issues or have questions, then don’t hesitate to ask for help in the comments section below the corresponding lesson.
Note: You can also reach out to the Real Python community on Slack or join live conversations during Office Hours, where you’ll have an opportunity to share your screen remotely.
Completing each task unlocks the next one. Once you’ve completed a task, you can compare your code with the sample solution provided in the following lesson. Remember that there’s often more than one way to solve a problem. If your solution differs slightly but meets the acceptance criteria and adheres to good programming practices, then that’s perfectly fine.
Good luck!
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
Python Software Foundation
2025 PSF Board Election Schedule
The PSF Board elections are a chance for the community to choose representatives to help the Python Software Foundation create a vision for and build the future of the Python community. This year, there are 4 seats open on the PSF Board. Check out who is currently on the board on the PSF's website. (Dawn Wages, Jannis Leidel, Kushal Das, and Simon Willison are at the end of their current terms.) As we previously shared, the Board election will take place a little later this year to better serve our community and ease pressure on PSF Staff.
Board Election Timeline
- Nominations open: Tuesday, July 29th, 2:00 pm UTC
- Nomination cut-off: Tuesday, August 12th, 2:00 pm UTC
- Announce candidates: Thursday, August 14th
- Voter affirmation cut-off: Tuesday, August 26th, 2:00 pm UTC
- Voting start date: Tuesday, September 2nd, 2:00 pm UTC
- Voting end date: Tuesday, September 16th, 2:00 pm UTC
Voting
You must be a Contributing, Supporting, or Fellow member by August 26th and affirm your intention to vote to participate in this election. If you are currently a Managing member, you will receive a communication soon notifying you that your membership type will be changed to Contributing per last year’s Bylaw change that merged Managing and Contributing memberships.
Check out the PSF membership page to learn more about membership classes and benefits. You can affirm your voting intention by following the steps in our video tutorial:
- Log in to psfmember.org
- Check your eligibility to vote (You must be a Contributing, Supporting, or Fellow member)
- Choose “Voting Affirmation” at the top right
- Select your preferred intention for voting in 2025
- Click the “Submit” button
Per another recent Bylaw change that allows for simplifying the voter affirmation process by treating past voting activity as intent to continue voting, if you cast your ballot last year, you will automatically be added to the 2025 voter roll. Please note that if you removed or changed your email on psfmember.org, you may not automatically be added to this year's voter roll.
If you have questions about membership, please email psf-elections@pyfound.org.
Run for the Board
Who runs for the board? People who care about the Python community, who want to see it flourish and grow, and also have a few hours a month to attend regular meetings, serve on committees, participate in conversations, and promote the Python community. Want to learn more about being on the PSF Board? Check out the following resources to learn more about the PSF, as well as what being a part of the PSF Board entails:
- Life as Python Software Foundation Director video
- FAQs About the PSF Board video
- Our past few Annual Impact Reports:
You can nominate yourself or someone else. We would encourage you to reach out to folks before you nominate them to make sure they are enthusiastic about the potential of joining the Board. Nominations open on Tuesday, July 29th, 2:00 pm UTC, so you have time to talk with potential nominees, research the role, and craft a nomination statement for yourself or others. Take a look at last year’s nomination statements for reference.
Learn more and join the discussion
You are welcome to join the discussion about the PSF Board election on the Discuss forum. This year, we’ll also be hosting PSF Board Office Hours on the PSF Discord in July and August to answer questions about running for and serving on the board. Subscribe to the PSF blog or, if you're a member, join the psf-member-announce mailing list to receive updates leading up to the election.
Talk Python to Me
#510: 10 Polars Tools and Techniques To Level Up Your Data Science
Are you using Polars for your data science work? Maybe you've been sticking with the tried-and-true Pandas? There are many benefits to Polars directly of course. But you might not be aware of all the excellent tools and libraries that make Polars even better. Examples include Patito which combines Pydantic and Polars for data validation and polars_encryption which adds AES encryption to selected columns. We have Christopher Trudeau back on Talk Python To Me to tell us about his list of excellent libraries to power up your Polars game and we also talk a bit about his new Polars course.<br/> <br/> <strong>Episode sponsors</strong><br/> <br/> <a href='https://talkpython.fm/agntcy'>Agntcy</a><br> <a href='https://talkpython.fm/sentry'>Sentry Error Monitoring, Code TALKPYTHON</a><br> <a href='https://talkpython.fm/training'>Talk Python Courses</a><br/> <br/> <h2 class="links-heading">Links from the show</h2> <div><strong>New Theme Song (Full-Length Download and backstory)</strong>: <a href="https://talkpython.fm/flasksong/" target="_blank" >talkpython.fm/blog</a><br/> <br/> <strong>Polars for Power Users Course</strong>: <a href="https://training.talkpython.fm/courses/polars-for-power-users" target="_blank" >training.talkpython.fm</a><br/> <strong>Awesome Polars</strong>: <a href="https://github.com/ddotta/awesome-polars?featured_on=talkpython" target="_blank" >github.com</a><br/> <strong>Polars Visualization with Plotly</strong>: <a href="https://docs.pola.rs/user-guide/misc/visualization/#plotly" target="_blank" >docs.pola.rs</a><br/> <strong>Dataframely</strong>: <a href="https://github.com/Quantco/dataframely?featured_on=talkpython" target="_blank" >github.com</a><br/> <strong>Patito</strong>: <a href="https://github.com/JakobGM/patito?featured_on=talkpython" target="_blank" >github.com</a><br/> <strong>polars_iptools</strong>: <a href="https://github.com/erichutchins/polars_iptools?featured_on=talkpython" target="_blank" >github.com</a><br/> <strong>polars-fuzzy-match</strong>: <a href="https://github.com/bnmoch3/polars-fuzzy-match?featured_on=talkpython" target="_blank" >github.com</a><br/> <strong>Nucleo Fuzzy Matcher</strong>: <a href="https://github.com/helix-editor/nucleo?featured_on=talkpython" target="_blank" >github.com</a><br/> <strong>polars-strsim</strong>: <a href="https://github.com/foxcroftjn/polars-strsim?featured_on=talkpython" target="_blank" >github.com</a><br/> <strong>polars_encryption</strong>: <a href="https://github.com/zlobendog/polars_encryption?featured_on=talkpython" target="_blank" >github.com</a><br/> <strong>polars-xdt</strong>: <a href="https://github.com/pola-rs/polars-xdt?featured_on=talkpython" target="_blank" >github.com</a><br/> <strong>polars_ols</strong>: <a href="https://github.com/azmyrajab/polars_ols?featured_on=talkpython" target="_blank" >github.com</a><br/> <strong>Least Mean Squares Filter in Signal Processing</strong>: <a href="https://www.geeksforgeeks.org/least-mean-squares-filter-in-signal-processing/?featured_on=talkpython" target="_blank" >www.geeksforgeeks.org</a><br/> <strong>polars-pairing</strong>: <a href="https://github.com/apcamargo/polars-pairing?featured_on=talkpython" target="_blank" >github.com</a><br/> <strong>Pairing Function</strong>: <a href="https://en.wikipedia.org/wiki/Pairing_function?featured_on=talkpython" target="_blank" >en.wikipedia.org</a><br/> <strong>polars_list_utils</strong>: <a href="https://github.com/dashdeckers/polars_list_utils?featured_on=talkpython" target="_blank" >github.com</a><br/> <strong>Harley Schema Helpers</strong>: <a href="https://tomburdge.github.io/harley/reference/harley/schema_helpers/?featured_on=talkpython" target="_blank" >tomburdge.github.io</a><br/> <strong>Marimo Reactive Notebooks Episode</strong>: <a href="https://talkpython.fm/episodes/show/501/marimo-reactive-notebooks-for-python#links-section" target="_blank" >talkpython.fm</a><br/> <strong>Marimo</strong>: <a href="https://marimo.io/?featured_on=talkpython" target="_blank" >marimo.io</a><br/> <strong>Ahoy Narwhals Podcast Episode Links</strong>: <a href="https://talkpython.fm/episodes/show/480/ahoy-narwhals-are-bridging-the-data-science-apis" target="_blank" >talkpython.fm</a><br/> <strong>Watch this episode on YouTube</strong>: <a href="https://www.youtube.com/watch?v=aIdvlJN1bNQ" target="_blank" >youtube.com</a><br/> <strong>Episode #510 deep-dive</strong>: <a href="https://talkpython.fm/episodes/show/510/10-polars-tools-and-techniques-to-level-up-your-data-science#takeaways-anchor" target="_blank" >talkpython.fm/510</a><br/> <strong>Episode transcripts</strong>: <a href="https://talkpython.fm/episodes/transcript/510/10-polars-tools-and-techniques-to-level-up-your-data-science" target="_blank" >talkpython.fm</a><br/> <br/> <strong>--- Stay in touch with us ---</strong><br/> <strong>Subscribe to Talk Python on YouTube</strong>: <a href="https://talkpython.fm/youtube" target="_blank" >youtube.com</a><br/> <strong>Talk Python on Bluesky</strong>: <a href="https://bsky.app/profile/talkpython.fm" target="_blank" >@talkpython.fm at bsky.app</a><br/> <strong>Talk Python on Mastodon</strong>: <a href="https://fosstodon.org/web/@talkpython" target="_blank" ><i class="fa-brands fa-mastodon"></i>talkpython</a><br/> <strong>Michael on Bluesky</strong>: <a href="https://bsky.app/profile/mkennedy.codes?featured_on=talkpython" target="_blank" >@mkennedy.codes at bsky.app</a><br/> <strong>Michael on Mastodon</strong>: <a href="https://fosstodon.org/web/@mkennedy" target="_blank" ><i class="fa-brands fa-mastodon"></i>mkennedy</a><br/></div>
June 17, 2025
PyCoder’s Weekly
Issue #686: Free-Threaded Update, GPU Programming, GitHub Actions, and More (June 17, 2025)
#686 – JUNE 17, 2025
View in Browser »
State of Free-Threaded Python
This is a blog post from the Python Language Summit 2025 giving an update on the progress of free-threaded Python. You may also be interested in the complete list of Language Summit Blogs.
PYTHON SOFTWARE FOUNDATION
GPU Programming in Pure Python
Talk Python interviews Bryce Adelstein Lelbach and they talk about using Python to harness the insane power of modern GPUs for data science and ML.
KENNEDY & LELBACH podcast
Making Friends with Agents: A Mental Model for Agentic AI
Explore a mental model to befriend your AI agent. This blog walks through designing goal-driven, tool-savvy agents that think in loops, speak your language, and bounce back from failure through durable execution →
TEMPORAL sponsor
Continuous Integration and Deployment Using GitHub Actions
Agile methodologies rely on robust DevOps systems to manage and automate common tasks in a continually changing codebase. GitHub Actions can help.
REAL PYTHON course
Python Jobs
Sr. Software Developer (Python, Healthcare) (USA)
Senior Software Engineer – Quant Investment Platform (LA or Dallas) (Los Angeles, CA, USA)
Causeway Capital Management LLC
Articles & Tutorials
A dict
That Can Report Which Keys Weren’t Used
When testing, you may want to make sure that all parts of a dictionary get accessed to get full coverage. This post shows a modified dict
that tracks which keys got used.
PETER BENGTSSON
Better Django Management Commands
Writing Django management commands can involve a ton of boilerplate code. This article shows you how to use two libraries that could cut your management command code in half: django-click and django-typer.
REVSYS
Easy-to-Deploy, Enterprise-Ready GenAI
Check out the Intel GenAI code library for ready-to-deploy and easy-to-integrate solutions.
INTEL CORPORATION sponsor
How Can You Structure Your Python Script?
Structure your Python script like a pro. This guide shows you how to organize your code, manage dependencies with PEP 723, and handle command-line arguments.
REAL PYTHON
Quiz: How Can You Structure Your Python Script?
In this quiz, you’ll test your understanding of organizing and structuring Python scripts. You’ll revisit key concepts about best practices for writing clear, maintainable, and executable Python code.
REAL PYTHON
Wyvern’s Open Satellite Feed
Wyvern is a satellite startup who has recently launched an open data program. This article plays with that data using Python libraries such as astropy, geocoder, rich and more.
MARKSBLOGG.COM
Pointblank: Data Validation Made Beautiful
This post introduces pointblank
a library for doing data validation. It includes chainable execution and interactive reports to see what is working in your data pipeline.
POSIT-DEV.GITHUB.IO
5 Non-LLM Software Trends to Be Excited About
Tired of reading about AI and LLMs? This post talks about other tech that is rapidly changing in the software world, including local-first applications, web assembly, the improvement of cross-platform tools, and more.
LEONARDO CREED
Concurrency in async
/await
and Threading
Want to write faster Python code? Discover the difference between async/await
and threading and how concurrency works in Python with real-world examples.
CHEUK TING HO
Defining Your Own Python Function
Learn how to define your own Python function, pass data into it, and return results to write clean, reusable code in your programs.
REAL PYTHON
TIL: HTML 404 Errors for FastHTML
A quick “Things I’ve Learned” post showing how to write a custom HTTP 404 handler for FastHTML.
DANIEL ROY GREENFIELD
PyData Virginia 2025 Talks
A list of the recorded talks from PyData Virginia 2025.
YOUTUBE.COM video
Projects & Code
Events
Weekly Real Python Office Hours Q&A (Virtual)
June 18, 2025
REALPYTHON.COM
PyData Bristol Meetup
June 19, 2025
MEETUP.COM
PyLadies Dublin
June 19, 2025
PYLADIES.COM
Python Nordeste 2025
June 20 to June 23, 2025
PYTHONNORDESTE.ORG
Python Coding Club for Teens (PyTahoua)
June 20 to June 23, 2025
PYTHONNIGER.ORG
Happy Pythoning!
This was PyCoder’s Weekly Issue #686.
View in Browser »
[ Subscribe to 🐍 PyCoder’s Weekly 💌 – Get the best Python news, articles, and tutorials delivered to your inbox once a week >> Click here to learn more ]
Adrarsh Divakaran
Will AI Replace Junior Developers? I Asked Experts at Pycon US